XML name of the component: filter
.
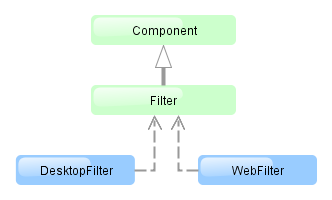
The component is implemented for Web Client and Desktop Client.
An example of component declaration in XML screen descriptor is shown below:
<dsContext> <collectionDatasource id="carsDs" class="com.company.sample.entity.Car" view="carBrowse"> <query> select c from ref$Car c order by c.createTs </query> </collectionDatasource> </dsContext> <layout> <filter id="carsFilter" datasource="carsDs"> <properties include=".*"/> </filter> <table id="carsTable" width="100%"> <columns> <column id="vin"/> <column id="model.name"/> <column id="colour.name"/> </columns> <rows datasource="carsDs"/> </table> </layout>
In the example above, a collectionDatasource
is defined in the dsContext
. The datasource selects Car
entity instances using JPQL query. The datasource which is to be filtered is specified in the filter
component’s datasource
attribute. Data is displayed using the Table component, which is connected to the same data source.
filter
may contain nested elements. They describe conditions available for user selection in dialog:
-
properties
– multiple entity attributes can be made available for selection. This element has the following attributes:Example:
<filter id="transactionsFilter" datasource="transactionsDs"> <properties include=".*" exclude="(masterTransaction)|(authCode)"/> </filter>
The following entity attributes are ignored when
properties
element is used:-
Collections (
@OneToMany
,@ManyToMany
). -
Attributes which do not have localized names.
-
The
version
attribute.
-
-
property
– explicitly includes an entity attribute by name. This element has the following attributes:-
name
– rrequired attribute, containing the name of entity attribute to be included. It can be a path (using ".") in the entity graph. For example:<filter id="transactionsFilter" datasource="transactionDs" applyTo="table"> <properties include=".*" exclude="(masterTransaction)|(authCode)"/> <property name="creditCard.maskedPan" caption="msg://EmbeddedCreditCard.maskedPan"/> <property name="creditCard.startDate" caption="msg://EmbeddedCreditCard.startDate"/> </filter>
-
caption
– localized entity attribute name displayed in filter conditions. Generally it is a string with themsg://
prefix in accordance with MessageTools.loadString() rules.If the
name
attribute is specified as an entity graph path (using ".") , thecaption
attribute is required. -
paramWhere
− specifies the JPQL expression which is used to select the list of condition parameter values if the parameter is a related entity. The{E}
placeholder should be used in the expression instead of the alias of the entity being selected.For example, let us assume that
Car
has a reference toModel
. Then possible condition parameter values list can be limited toAudi
models only:<filter id="carsFilter" datasource="carsDs"> <property name="model" paramWhere="{E}.manufacturer = 'Audi'"/> </filter>
Screen parameters, session attributes and screen components including those showing other parameters can be used in JPQL expression. Query parameters specification rules are described in Section 4.5.3.2, “CollectionDatasourceImpl Queries”.
An example of session and screen parameters usage is shown below:
{E}.createdBy = :session$userLogin and {E}.name like :param$groupName
With the
paramWhere
clause, you can introduce dependencies between parameters. For example, let us assume thatManufacturer
is a separate entity. That isCar
has a reference toModel
which in turn has a reference toManufacturer
. Then you may want to create two conditions for the Cars filter: first to select a Manufacturer and second to select a Model. To restrict the list of models by previously selected manufacturer, add a parameter to theparamWhere
expression:{E}.manufacturer.id = :component$filter.model_manufacturer90062
The parameter references a component which displays Manufacturer parameter. You can see the name of the component showing condition parameter by opening context menu on a condition table row in the filter editor:
-
paramView
− specifies a view, which will be used to load the list of condition parameter values if the parameter is a related entity. For example,_local
. If view is not specified,_minimal
view will be used.
-
-
custom
– the element defining an arbitrary condition. The element content should be a JPQL expression (JPQL Macros can be used), which will be added to the data source query'swhere
clause. The{E}
placeholder should be used in the expression instead of the alias of the entity being selected. The condition can only have one parameter denoted by "?" if used.An example of a filter with arbitrary conditions is shown below:
<filter id="carsFilter" datasource="carsDs"> <properties include=".*"/> <custom name="vin" paramClass="java.lang.String" caption="msg://vin"> {E}.vin like ? </custom> <custom name="colour" paramClass="com.company.sample.entity.Colour" caption="msg://colour" inExpr="true"> ({E}.colour.id in (?)) </custom> <custom name="repair" paramClass="java.lang.String" caption="msg://repair" join="join {E}.repairs cr"> cr.description like ? </custom> <custom name="updateTs" caption="msg://updateTs"> @between({E}.updateTs, now-1, now+1, day) </custom> </filter>
custom
conditions are displayed in the Custom conditions section of the dialog:custom
attributes:-
caption
− required attribute, localized condition name. Generally it is a string with themsg://
prefix in accordance with MessageTools.loadString() rules. -
paramClass
− Java class of the condition parameter. If the parameter is not specified, this attribute is optional. -
inExpr
− should be set totrue
, if the JPQL expression containsin (?)
conditions. In this case user will be able to enter several condition parameter values. -
join
− optional attribute. It specifies a string, which will be added to the data source queryfrom
section. This can be required to create a complex condition based on an attribute of a related collection.join
orleft join
statements should be included into the attribute value.For example, let us assume that the
Car
entity has arepairs
attribute, which is a related entityRepair
instances collection. Then the following condition can be created to filterCar
byRepair
entity'sdescription
attribute:<filter id="carsFilter" datasource="carsDs"> <custom name="repair" caption="msg://repair" paramClass="java.lang.String" join="join {E}.repairs cr"> cr.description like ? </custom> </filter>
If the condition above is used, the original data source query
select c from sample$Car c order by c.createTs
will be transformed into the following one:
select c from sample$Car c join c.repairs cr where (cr.description like ?) order by c.createTs
-
paramWhere
− specifies a JPQL expression used to select the list of condition parameter values if the parameter is a related entity. See the description of theproperty
element's attribute of the same name. -
paramView
− specifies a view, which will be used when a list of condition parameter values are loaded if the parameter is a related entity. For example,_local
. If it is not specified,_minimal
view will be used.
filter
attributes:
-
editable
– if the attribute value isfalse
, the option is disabled. -
required
– if the attribute value istrue
, user should select one of available filters. If no default filter is set for the screen, the first created filter will be automatically selected in the filter list. -
manualApplyRequired
− defines when the filter will be applied. If the attribute value isfalse
, the filter will be applied when the screen is opened. If the value istrue
, the filter will be applied only after the button is clicked. This attribute takes precedence over the cuba.gui.genericFilterManualApplyRequired application property. -
useMaxResults
− limits the page size of entity instances loaded into the data source. It is set totrue
by default.If the attribute value is
false
, the filter will not show the Show rows field. The number of records in the data source (and displayed in the table accordingly) will be limited only by theMaxFetchUI
parameter of theentity statistics, which is set to 10000 by default.If the attribute is not specified or is
true
, the Show rows field will be displayed only if the user has specificcuba.gui.filter.maxResults
permission. If thecuba.gui.filter.maxResults
permission is not granted, the filter will force selecting only the first N rows without user to be able to disable it or specify another N. N is defined byFetchUI
,DefaultFetchUI
parameters. They are obtained from the entity statistics mechanism.A filter shown below has the following parameters:
useMaxResults="true"
, specific permission is denied, andcuba.gui.filter.maxResults
DefaultFetchUI = 2.
-
textMaxResults
- enables using the text field instead of the drop-down list as the Show rows field.false
by default. -
folderActionsEnabled
− if it is set tofalse
, the following filter actions will be hidden: Save as Search Folder, Save as Application Folder. By default, the attribute value istrue
, and Save as Search Folder, Save as Application Folder are available. -
applyTo
− optional attribute, contains the identifier of a component associated with the filter. It is used when access to related component views is required. For example, when saving the filter as a search folder or as an application folder, the view that will be applied when browsing this folder can be specified. -
caption
- allows setting a custom caption for the filter panel. -
columnsQty
- defines the number of columns for conditions on the filter panel. Default value is 3.
All filter
attributes:
applyTo | datasource | folderActionsEnabled | margin |
caption | editable | id | required |
columnsQty | enable | manualApplyRequired | stylename |
useMaxResults | visible |
filter
elements:
properties
attributes:
property
attributes:
custom
attributes: