The FileUploadField
component allows a user to upload files to a server. The component is a
button; when it is clicked, the screen will show a standard OS file picker window where you can select one file.
To allow a user to upload multiple files, use FileMultiUploadField.
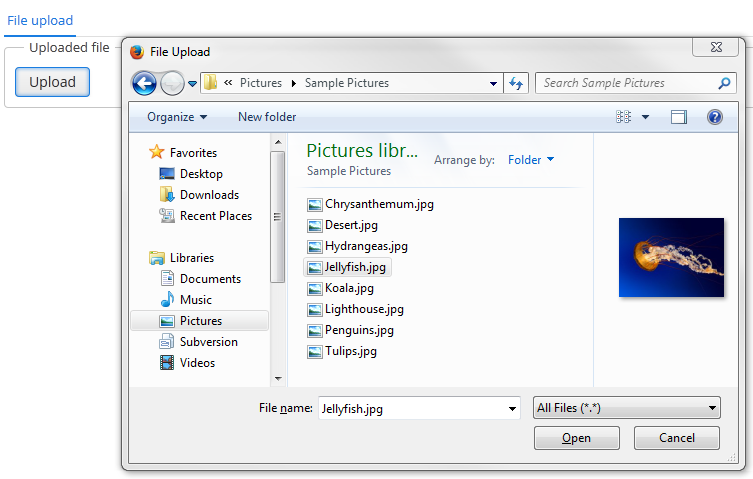
XML name of the component: upload
.
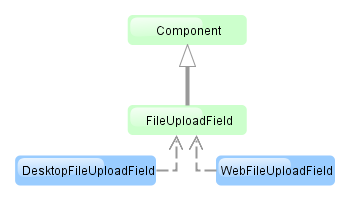
The component is implemented for Web Client and Desktop Client.
Below is an example of using the component.-
Declare the component in an XML screen descriptor:
<upload id="uploadField" caption="msg://upload"/>
-
In the screen controller, inject the component itself, and the FileUploadingAPI and DataSupplier interfaces. Then, in
init()
add a listener to the component, which will react on successful upload events or errors:@Inject protected FileUploadField uploadField; @Inject protected FileUploadingAPI fileUploading; @Inject protected DataSupplier dataSupplier; @Override public void init(Map<String, Object> params) { uploadField.addListener(new FileUploadField.ListenerAdapter() { @Override public void uploadSucceeded(Event event) { FileDescriptor fd = uploadField.getFileDescriptor(); try { // save file to FileStorage fileUploading.putFileIntoStorage(uploadField.getFileId(), fd); } catch (FileStorageException e) { throw new RuntimeException(e); } // save file descriptor to database dataSupplier.commit(fd, null); showNotification("File uploaded: " + uploadField.getFileName(), NotificationType.HUMANIZED); } @Override public void uploadFailed(Event event) { showNotification("File upload error", NotificationType.HUMANIZED); } }); }
The component will invoke
uploadSucceeded()
after uploading the file to a temporary storage of the client tier. At this point, you can get component’sFileDescriptor
object, which corresponds to the uploaded file.com.haulmont.cuba.core.entity.FileDescriptor
(do not confuse withjava.io.FileDescriptor
) is a persistent entity, which uniquely identifies an uploaded file and then is used to download the file from the system.FileUploadingAPI.putFileIntoStorage()
method is used to move the uploaded file from the temporary client level storage to FileStorage. Parameters of this method are temporary storage file identifier and theFileDescriptor
object. Both of these parameters are provided byFileUploadField
.After uploading the file to
FileStorage
theFileDescriptor
instance is saved in a database by invokingDataSupplier.commit()
. The saved instance returned by this method can be set to an attribute of an entity related to this file. In this case,FileDescriptor
is simply stored in the database and gives access to the file through the -> screen.uploadFailed()
will be invoked by theFileUploadField
component if an error occurs when uploading a file to the temporary storage of the client level. -
Maximum upload size is determined by the cuba.client.maxUploadSizeMb application property and is equal to 20Mb by default. If a user selects a file of a larger size, a corresponding message will be displayed and the upload will be interrupted.
upload
attributes: